Implement authentication using Supabase
-- 2 min read
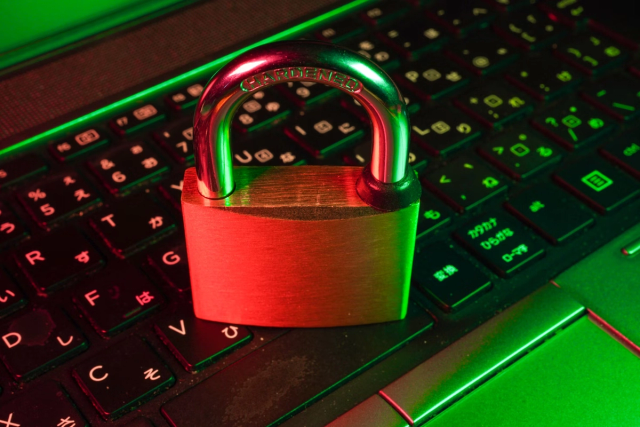
When using a website, some information might only be available to people that match a certain criteria, e.g a student in an institution. In order to access this information, one needs to prove they are who they say they are, usually by providing a username and password. This process is called authentication.
How do I do it?
Authentication is fairly easy to implement using Supabase. Supabase is a service that provides a hosted database (postgres), authentication, amongst other services. It handles all the heavy lifting and provides you with client a library that you can use in your favourite programming language. This client library provides a simple api for authentication and interacting with your database .
Project setup
We will be using Remix framework in these examples. Let's set up our project. Initialize a Remix app with the following command:
npx create-remix@latest
Setting up Supabase in a Remix application is a straightforward process. Follow the steps here to create a Supabase client.
Signup
The first step in an authentication flow is signing up users. Let's set up a sign up page with a form to capture the user's details.
// /routes/signup.jsx
export default function Signup() {
return (
<main>
<h1>Signup</h1>
<form method="post">
<fieldset>
<label>
Email:
<input type ="email" name="email" />
</label>
<label>
Password:
<input type="password" name="password" />
</label>
<button type="submit">Sign up</button>
</fieldset>
</form>
</main>
);
}
When a user submits the form, we get access to the data they submitted in the action function in the signup route.
// routes/signup.jsx
import { createClient } from "../.server/supabase";
export async function action({ request }) {
let formData = await request.formData();
let email = formData.get('email');
let password = formData.get('password');
// Sign up using Supabase
let { supabase, headers } = createClient(request);
let { data, error } = await supabase.auth.signUp({ email, password });
return null;
}
// Rest of your code
The user will get an email that they should click to verify their email.
That was quite easy, now let's see how to log in a user.
Login
To log in a user we just need a form to collect their user name and password and check it against Supabase to see if they are a valid user. Let's create our login page with a form and an action function to handle the form submission.
// /routes/login.jsx
import { redirect } from '@remix-run/react';
import { createClient } from "../.server/supabase";
export async function action({ request }) {
let formData = await request.formData();
let email = formData.get('email');
let password = formData.get('password');
// Log in with Supabase
let { data, error } = await supabase.auth.signInWithPassword({ email, password });
return redirect('/');
}
export default function Signup() {
return (
<main>
<h1>Login</h1>
<form method="post">
<fieldset>
<label>
Email:
<input type ="email" name="email" />
</label>
<label>
Password:
<input type="password" name="password" />
</label>
<button type="submit">Log in</button>
</fieldset>
</form>
</main>
);
}
All we need to do is pass the email and password that the user provided to Supabase using the function signInWithPassword()
That's it 👋🏾